How to Get Current Working Directory in Python 3
In this tutorial, we are going to learn how to get current working directory in the Python 3 programming language.
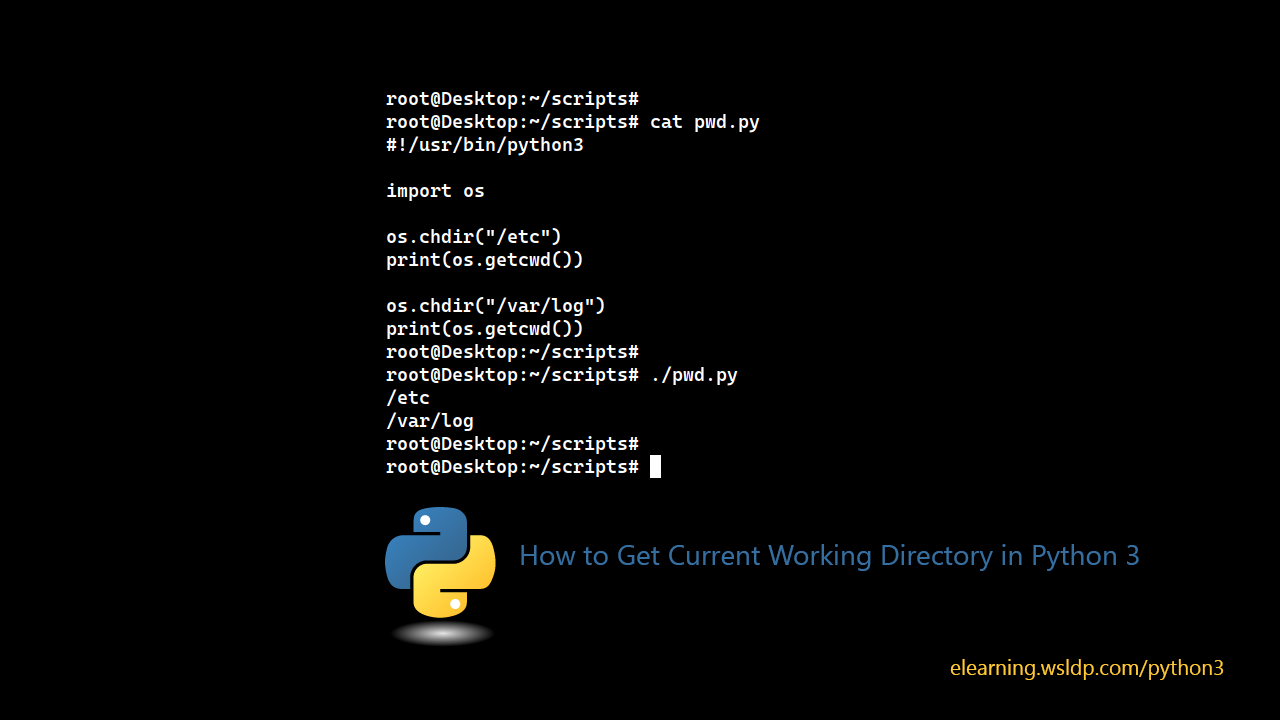
In Python 3 we can get the current working directory using os.getcwd()
function. The getcwd()
function is part of the os
module, so first of all, we need to import the python 3 os
module.
import os
Then we can use the os.getcwd()
function to print the working directory.
import os
print(os.getcwd())
The above code will print the location where your python script is currently running.
Also, we can store the current working directory in a variable instead of printing it straight away.
import os
cwd = os.getcwd()
print(cwd)
Change Working Directory in Python 3
To change the working directory in python 3, we use the os.chdir()
function (remember, you have to import the os
module first).
os.chdir(path)
Example 1
import os
os.chdir("/etc")
print(os.getcwd())
os.chdir("/var/log")
print(os.getcwd())
Above Example will output the following:
/etc
/var/log
Example 2 : Microsoft Windows
import os
os.chdir("C:\Windows\System32\drivers\etc")
print(os.getcwd())
The above Python code will output following in microsoft Windows:
C:\Windows\System32\drivers\etc
Python getcwd()
function can be used in both Linux and Windows operating systems to get current working directory.