How to Convert List to String in Python
Many times you will need to convert a list to a string. To do that in Python, we use the .join()
method. It also allows you to specify the separator.
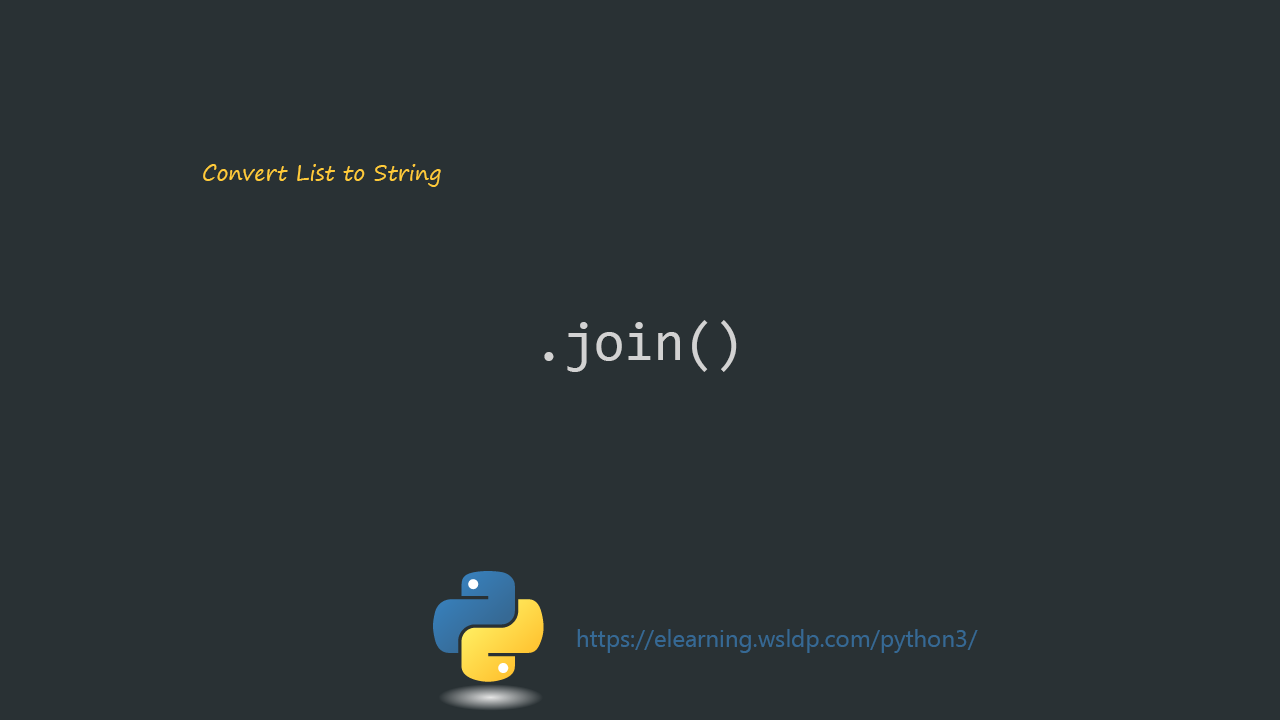
The syntax for converting a list to string using the join()
method is as follows:
''.join(list)
Here is an example code to demonstrate this:
colors = ['red', 'green', 'blue']
string = ''.join(colors)
print(string)
In this example, we have a variable called colors that contains three objects. On the next line, we convert the colors list to a string and store it in a new variable.
We get the following output for the preceding Python code:
redgreenblue
We left the separator blank so that each element of the list is joined without any separating characters.
Two quotes(”) before the join function is for separating characters
In the previous example, we didn’t use any separating characters. Therefore the list is converted to one word.
If we put a space in there, the join()
function will add a space between each list item, as shown in the following example:
colors = ['red', 'green', 'blue']
string = ' '.join(colors)
print(string)
If we run the preceding code, we get the following output:
red green blue
Here is another example of a code block that converts a list to a string:
colors = ['red', 'green', 'blue']
delimiter = ", "
string = delimiter.join(colors)
print(string)
In the above example, we have a variable called delimiter whose value is set to a comma and space. On the next line, we use the delimiter variable with the join()
method to create a comma-separated string.
When we run this, we get the following:
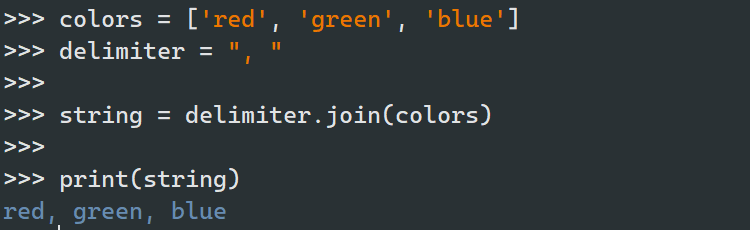
More Examples with Lists Slicing
Convert a list of integers with a hyphen (-) as the separator:
integers = ['1', '2', '3', '4', '5']
string = '-'.join(integers)
Convert list to a string in reverse order:
integers = ['1', '2', '3', '4', '5']
string = ''.join(integers[::-1])
Take the first three elements and convert them to a string with a comma as the separator:
integers = ['1', '2', '3', '4', '5']
string = ','.join(integers[0:3])
Take the first item and convert it to a string:
integers = ['1', '2', '3', '4', '5']
string = ''.join(integers[0])
Take the last item on the list and convert it to a string:
integers = ['1', '2', '3', '4', '5']
string = ''.join(integers[-1])
Summary
- In Python 3, we use the
join()
method to convert a list to a string. - The
join()
method can be used to create a string where the individual elements are separated by a given separator character.