How to Convert String to List in Python
In this tutorial, you will learn how to convert string to list in Python programming.
In python 3, the list()
function converts a string to a list. The syntax of the list()
function is as follows:
list('string')
The list function takes a string as an argument. Following is an example:
my_list = list('Hello World')
print(my_list)
In the above example, we converted the string ‘Hello World’ to a Python list and stored it in a new variable called my_list
.
The list function takes a string as its argument and converts each character in that string (including spaces) to a list item.
Following is another string to list example:
hello = 'Hello World'
my_list = list(hello)
print(my_list)
In this example, we have a string variable called hello. We converted it to a list and stored it in a new variable called my_list
. The original string variable remains the same.
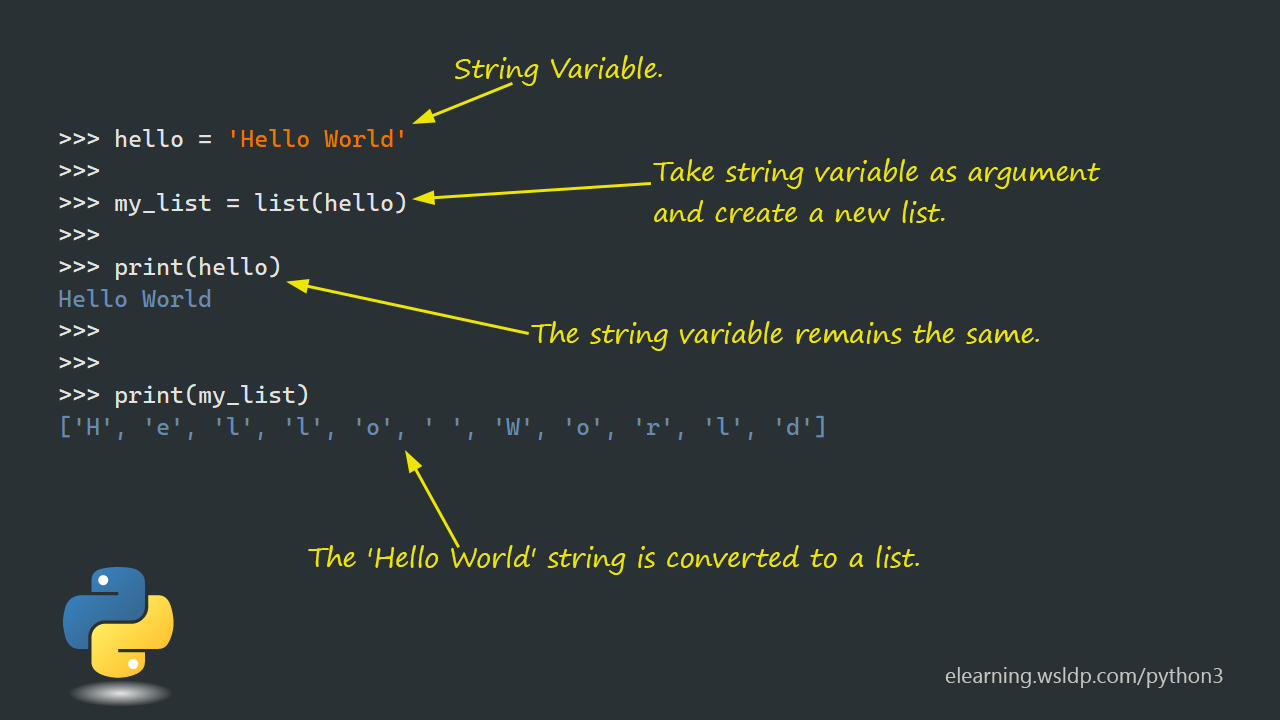
Splitting a string
You can use the split()
method and specify a character you want to treat as the separator (delimiter).
In the following example, we will use the split method to convert a comma-separated string into a list.
string = "1,2,3,4,5"
my_list = string.split(',')
print(my_list)
The result of the split is a list:
['1', '2', '3', '4', '5']
String to List Examples with String Slicing
Get the first character of the string and convert it to a list:
hello = 'Hello World'
my_list = list(hello[0])
Get the last character and convert it to a Python list:
hello = 'Hello World'
my_list = list(hello[-1])
Convert string to a list in reverse order:
hello = 'Hello World'
my_list = list(hello[::-1])
Extract the first five characters (From 0 index up to, but not including the fifth index) and convert them to a list:
hello = 'Hello World'
my_list = list(hello[0:5])
Get the last five characters and convert them to a Python list:
hello = 'Hello World'
my_list = list(hello[-1:-6:-1])
Extract and convert every third letter up to (but not including) index location 8:
hello = 'Hello World'
my_list = list(hello[:8:3])