How To Delete a List in Python
We can use the del
function to delete a list in Python. The following example shows how it works:
numbers = [1, 2, 3, 4, 5]
del numbers
The del
method completely removes the list from memory. Therefore the list will no longer be accessible. If you try to access the list, you will get an error like NameError: name 'num' is not defined
.
The del
command can also be used to remove individual list items from the list by index.
The following code deletes the first element:
numbers = [1, 2, 3, 4, 5]
del numbers[0]
If you want to delete the items of a list, but not the list itself, use the clear()
function.
numbers = [1, 2, 3, 4, 5]
numbers.clear()
The clear()
function removes all items from the list, and the list becomes an empty list, but the list is still accessible.
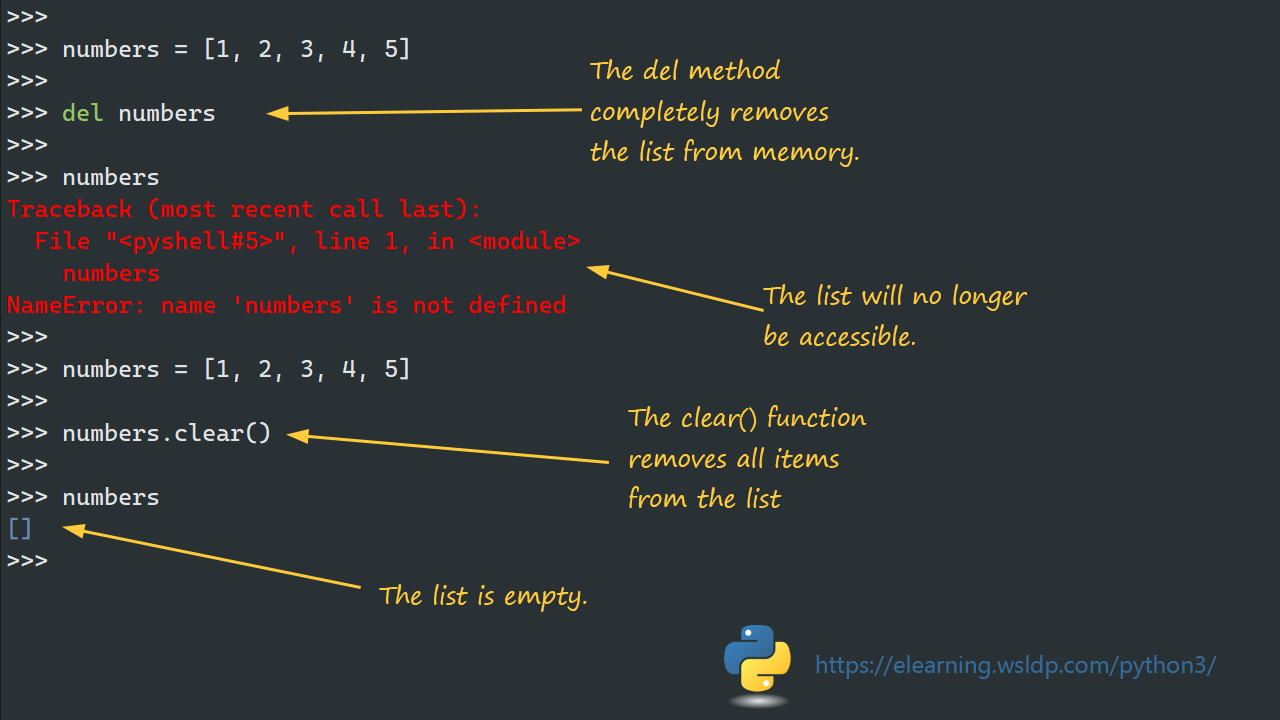