How To Get the Last Element of a List in Python
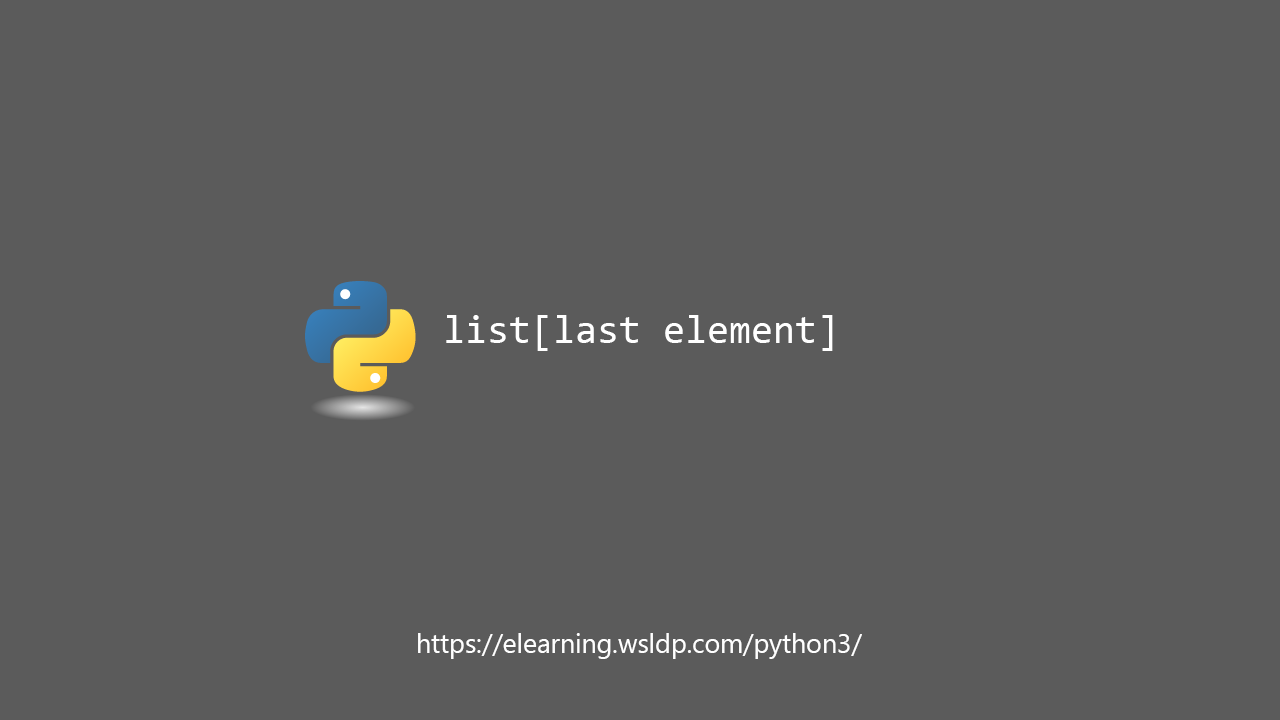
Python lists support negative index values. The negative one (-1) is always the last element of the list.
With that in mind, to get the last element of a list in python, we use the square bracket notation as follows:
list[-1]
The following Python example shows how it works:
colors = ['white','red', 'green', 'blue']
last_element = colors[-1]
print(last_element)
Negative index values count from right to left, where -1
is the last index, -2
is the second-to-last index, and so on.
The following example shows how to get the element before the last one:
colors = ['white','red', 'green', 'blue']
print(colors[-2])
In Python, using 0
as the index value is always guaranteed to return the last element in the list and -1
always returns the last element in the list.