How To Convert Python List to Comma Separated String
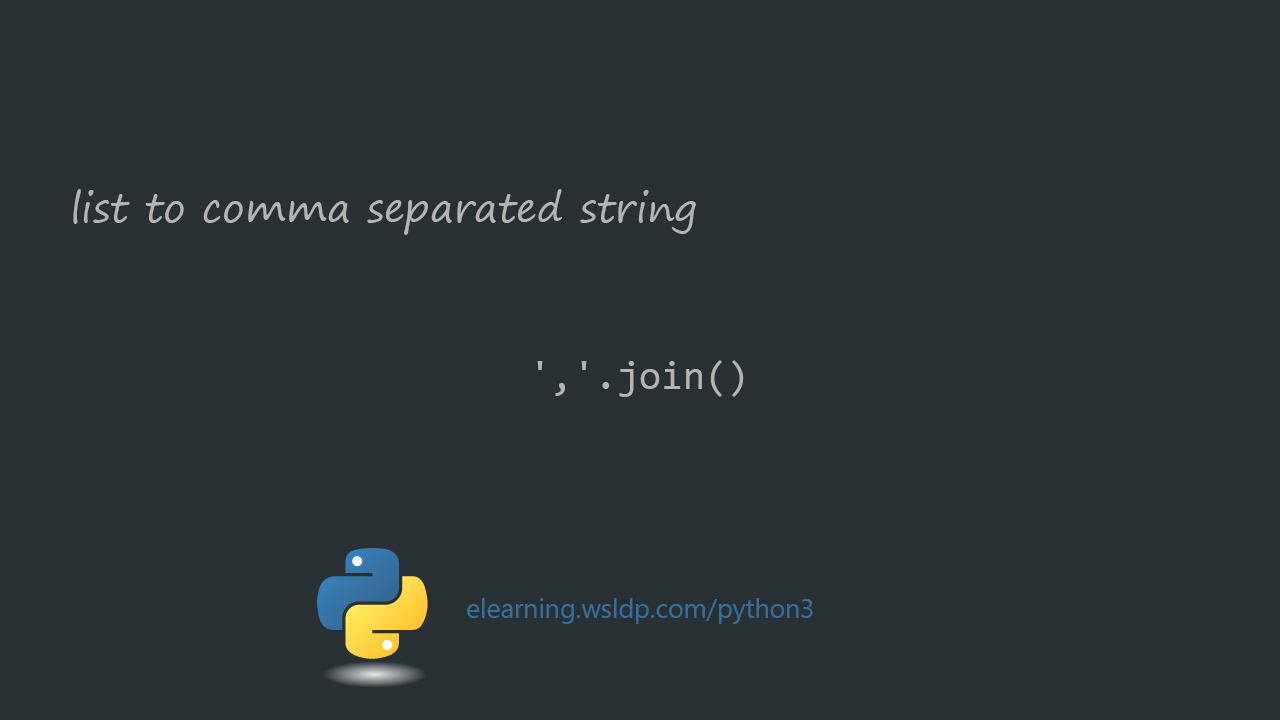
To convert a Python list to a comma-separated string, you will want to use the join()
method.
Here is an example code to demonstrate this:
letters = ['a', 'b', 'c', 'd']
string = ','.join(letters)
print(string)
After running the preceding code, we get the following output:
a,b,c,d
The join()
method creates a string where the individual list items are separated by a given separator character.
You can use any character(s) as the separator. For example, in the following code, we use a comma and space to separate each list item.
letters = ['a', 'b', 'c', 'd']
string = ', '.join(letters)
print(string)
When we run this, we get the following:
a, b, c, d
If the list contains integers, you need to convert each integer to a string before converting. For that, you can use a list comprehension, as shown in the following example:
numbers = [1, 2, 3, 4, 5]
string = ','.join([str(number) for number in numbers])
print(string)
Here is another example in which we use a variable to hold the separator:
letters = ['a', 'b', 'c', 'd']
separator = ","
string = separator.join(letters)