How to add pause delay in python using sleep function
In this tutorial we are going to learn how to pause python script using time.sleep()
function.
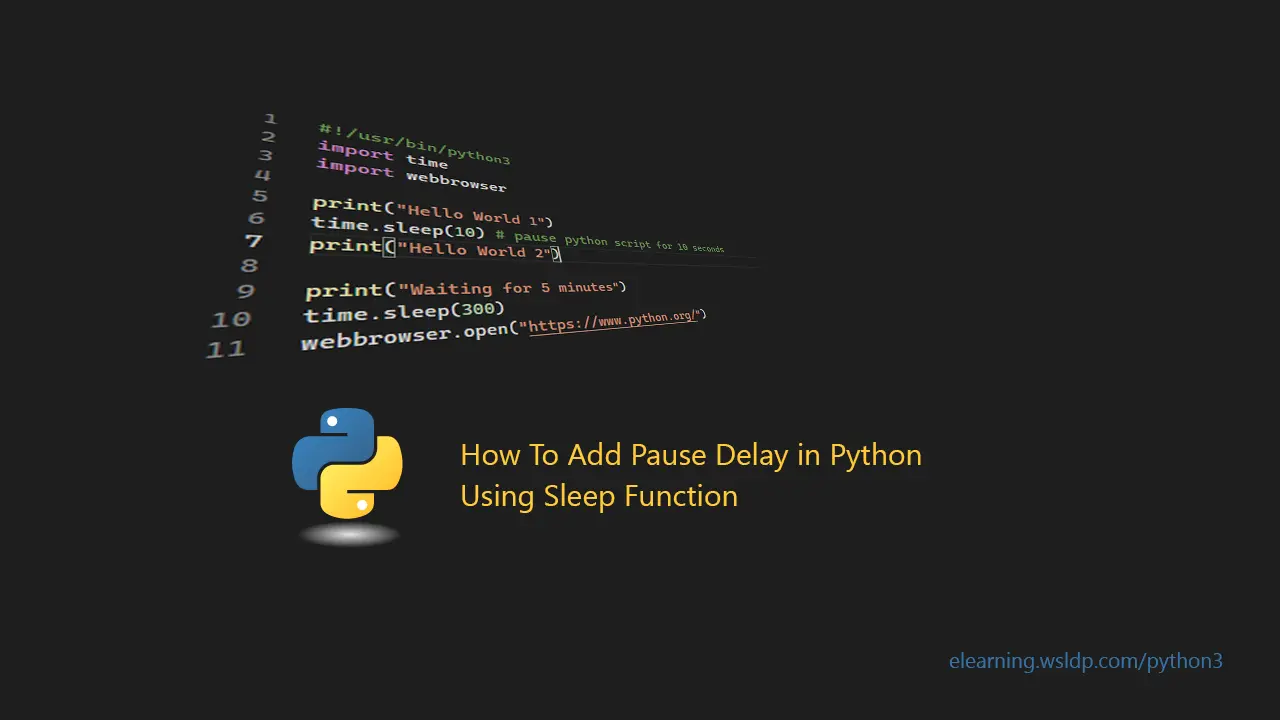
Often you will need to add a delay to python scripts, which means you pause the execution of the python script for a number of seconds before executing the next line of code.
We can add delay to a python script using the time module. The sleep()
function of the time
module pauses your python program for the specified number of seconds.
#!/usr/bin/python3
import time
time.sleep(n) # n = number of seconds for wait
Example 1
#!/usr/bin/python3
import time
print("Hello World 1")
time.sleep(10) # pause python script for 10 seconds
print("Hello World 2")
In the above example, first, we print the “Hello World 1”, then we pause the python script for 10 second (Add 10 second delay). After 10 seconds it will print the “Hello World 2”.
Example 2 – Open web browser after delay
import time
import webbrowser
print("Waiting for 5 minutes")
time.sleep(300)
webbrowser.open("https://www.python.org/")
In the above example, first, we print the message “Waiting for 5 minutes”, then we pause the python script for 5 minutes (300 seconds). After 5 minutes of delay, it will open the web browser with the URL https://www.python.org/.
So that is how we can pause and add delay in python 3 using time.sleep()
function.