Learn How to Append Items to Python List
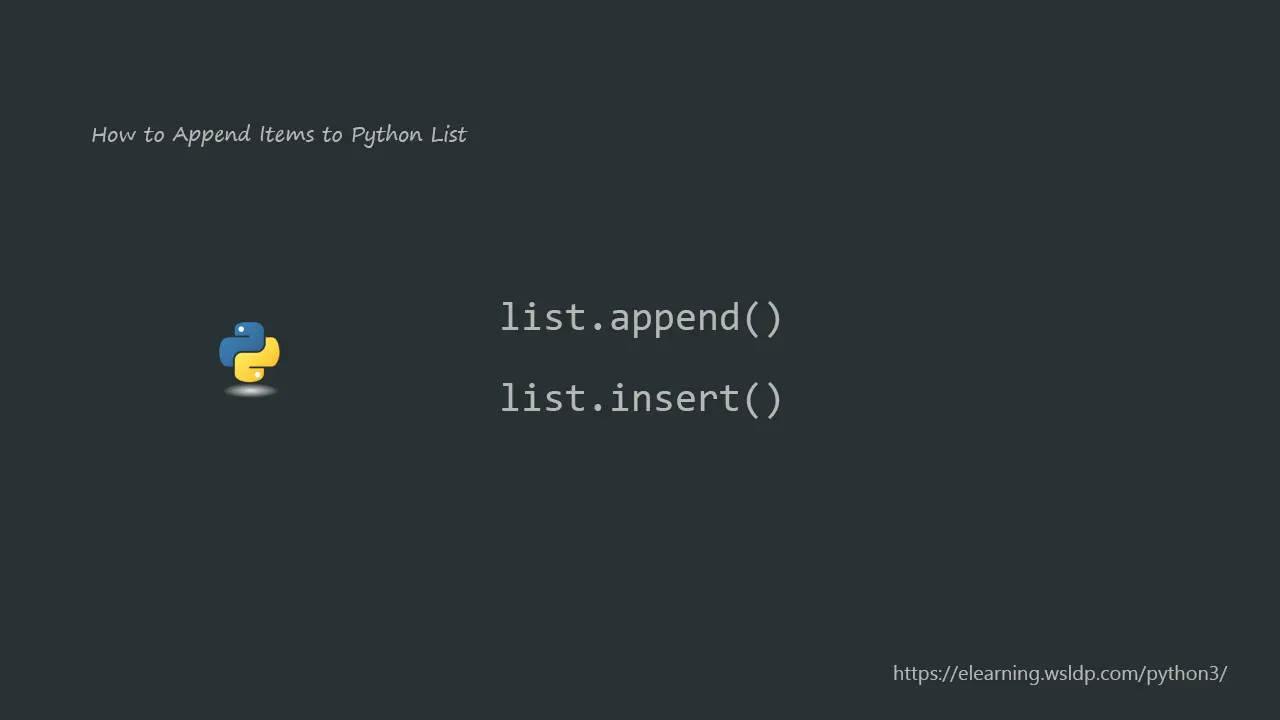
In this Python 3 tutorial, we will learn how to append new elements to a python list using the Python append()
function.
Add elements to the end of a list
In Python, the append()
method is used to append items to a python list. When you append an item to a list, the new item will be added to the end of the python list.
list.append("new item")
Example 1
In this example, we have a Python list called languages, which contains a list of programming languages.
languages = ["java", "php", "javascript", "perl"]
Then, we add the new element “python” to the end of the list using the append()
method.
languages = ["java", "php", "javascript", "perl"]
print(languages)
languages.append("python")
print(languages)
The above code will append python to the languages list. The code will output the following.
['java', 'php', 'javascript', 'perl']
['java', 'php', 'javascript', 'perl', 'python']
Insert Elements into a list by index position
Instead of appending items to the end of the list, we can use the insert()
method to add items at any given position by index number (remember that Python lists start with index 0).
Example 2
languages = ["java", "php", "javascript", "perl"]
print(languages)
languages.insert(0, "python")
print(languages)
In this example, we insert “python” at the beginning of the languages list(since 0 is the first index position).
['java', 'php', 'javascript', 'perl']
['python', 'java', 'php', 'javascript', 'perl']
Example 3
languages = ["java", "php", "javascript", "perl"]
print(languages)
languages.insert(2, "python")
print(languages)
In this example, we insert “python” to the index position 2, the third element of the languages list. As a result, “javascript” and “perl” will shift one position to the right.
['java', 'php', 'javascript', 'perl']
['java', 'php', 'python', 'javascript', 'perl']
Summary – Python Append to List
- In python 3, the
append()
method adds items to the end of the list. - The Python
insert()
function inserts elements at any position by the index of the new element.