Learn How to Check if a String Is a Number in Python 3
In this tutorial, we will learn how to check if a string is a number in the Python 3 programming language.
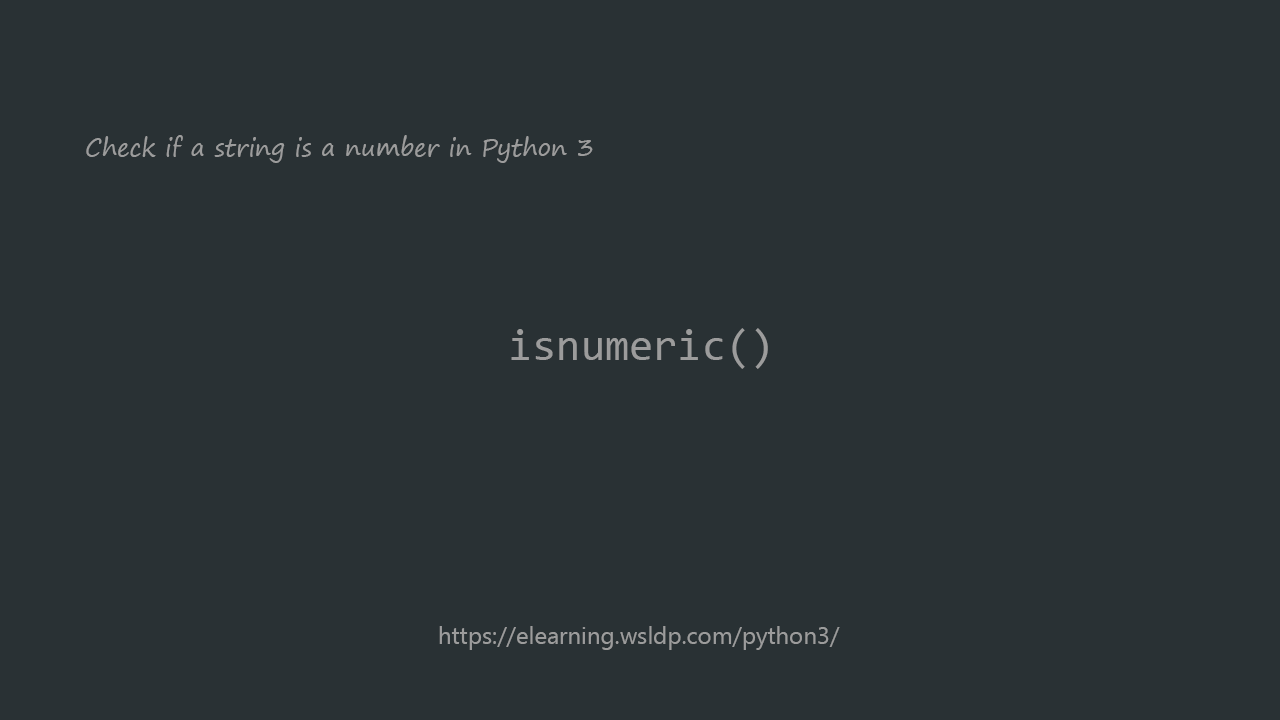
Python 3 has a built-in string function called str.isnumeric()
which will check whether a string is numeric or not. However, the str.isnumeric()
function will return False
for floating-point numbers. To check if a string is a float, we can use a try-except statement.
Python is string numeric
Python str.isnumeric()
function will return True
if the given string is numeric or return False
otherwise.
Example 1
print(str.isnumeric("10"))
The Above python code will return True
since “10” is a numeric value.
True
Example 2
print(str.isnumeric("abc"))
The Above python code will return False
.
We can also use the isnumeric()
function with a string variable as follows.
number = "100"
print(number.isnumeric())
To return True
, the whole string has to be numbers only, or it will return False
. For Example, the following code will return False
since the variable number contains the letter “A”.
number = "100A"
print(number.isnumeric())
Check if a string is a Float in Python 3
Python str.isnumeric()
function will return False
for the floating-point numbers. But we can write a simple function using the try-except
statement to check whether a string is a floating-point number or not.
def is_number(string):
try:
float(string)
return True
except ValueError:
return False
As per the above python code, we created a new function called is_number()
. The Function will simply try to convert the given string to a float. If the string can be converted to float, then the is_number function will return True
.
Example
def is_number(string):
try:
float(string)
return True
except ValueError:
return False
print(is_number("10.5"))
The Above Python Code will return True
.
Summary
In this tutorial, we learned how to check if a string is a number in python programming.
- We used the python
str.isnumeric()
function to check whether a string is numeric or not. - To check Floating point numbers, we wrote a simple python function called
is_number()
.