How To Clear List in Python 3, Remove All Items From List
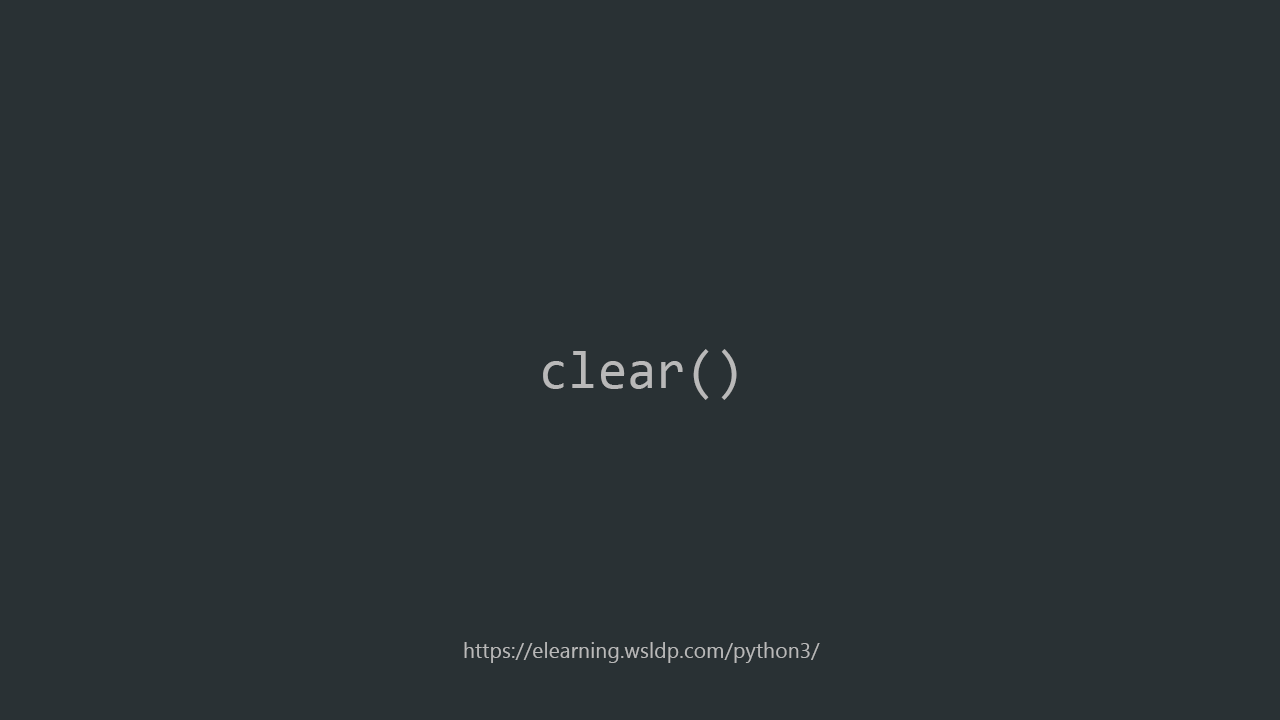
To clear a list in Python, you will want to use the clear()
function. Clearing a list means removing all items in the list.
The following example demonstrates how to use the clear()
function to remove all elements from a Python List:
colors = ['red', 'green', 'blue']
colors.clear()
print(len(colors))
In this example, we have a list called colors which contains three items. In the second line, we clear the colors list using the clear()
function.
In the last line of code, we check the length of the list using the len() function. When we run this Python script, it should return 0 because now the list is empty.
An alternative to the clear()
method would be the del
function with the square bracket notation.
colors = ['red', 'green', 'blue']
del colors[:]
print(len(colors))
If you want to delete a list (remove the variable from memory), use the del
statement without using the square brackets, as follows:
colors = ['red', 'green', 'blue']
del colors
The del
function completely removes a variable from memory.