How to Create Empty List in Python
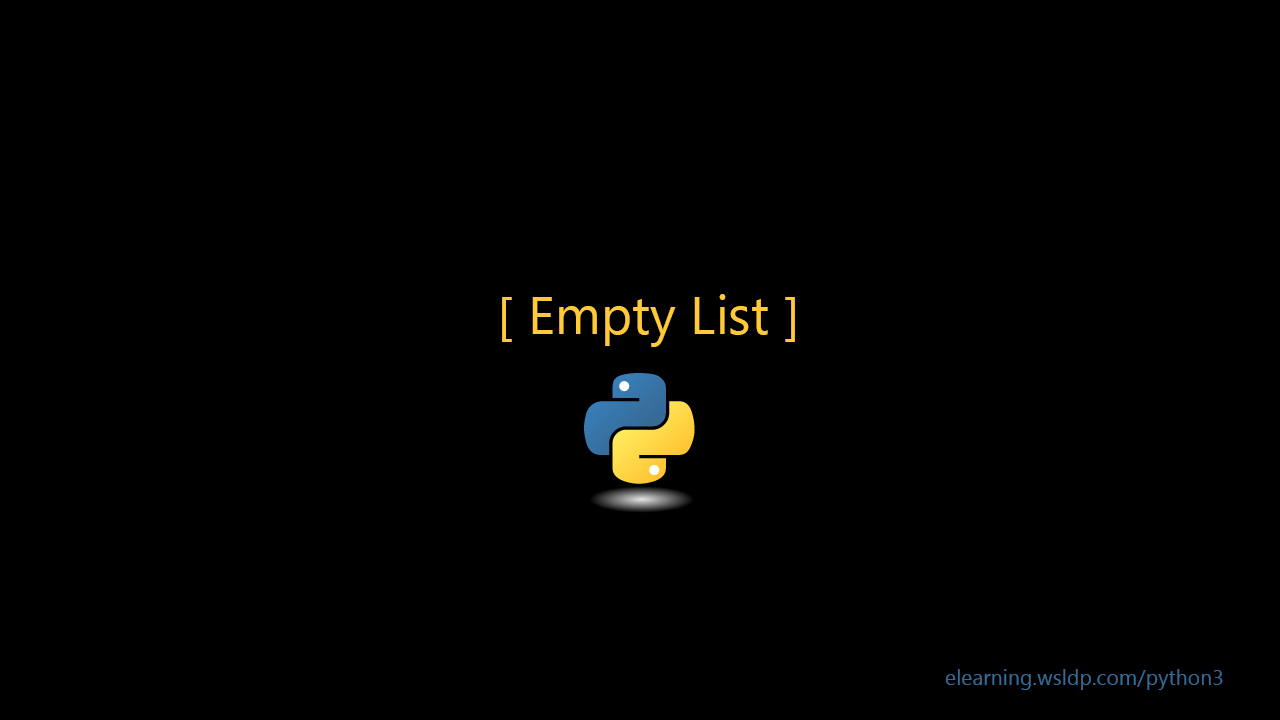
In Python 3, we create an empty list by assigning two square brackets []
to a variable:
variable_name = []
Following is an example of an empty list in Python 3:
country_list = []
In the example, the variable country_list
is an empty list. We can use Python’s built-in len
function to check the length of the list.
country_list = []
print(len(country_list))
If you want, you can create a list and assign elements at the same time, as shown in the following example:
country_list = ['France', 'USA', 'Russia', 'China']
You Can Add Elements to Empty List With .append() Function
We can add elements to a list as the code executes, using the .append()
method:
country_list = []
print(country_list)
country_list.append('UK')
country_list.append('Canada')
print(country_list)
The append()
method adds an object at the end of the list. To add an element to a specific position use the insert()
function:
country_list = []
print(country_list)
country_list.append('UK')
country_list.append('Canada')
print(country_list)
country_list.insert(0, "Spain")
print(country_list)