How to get Current Date in Python 3
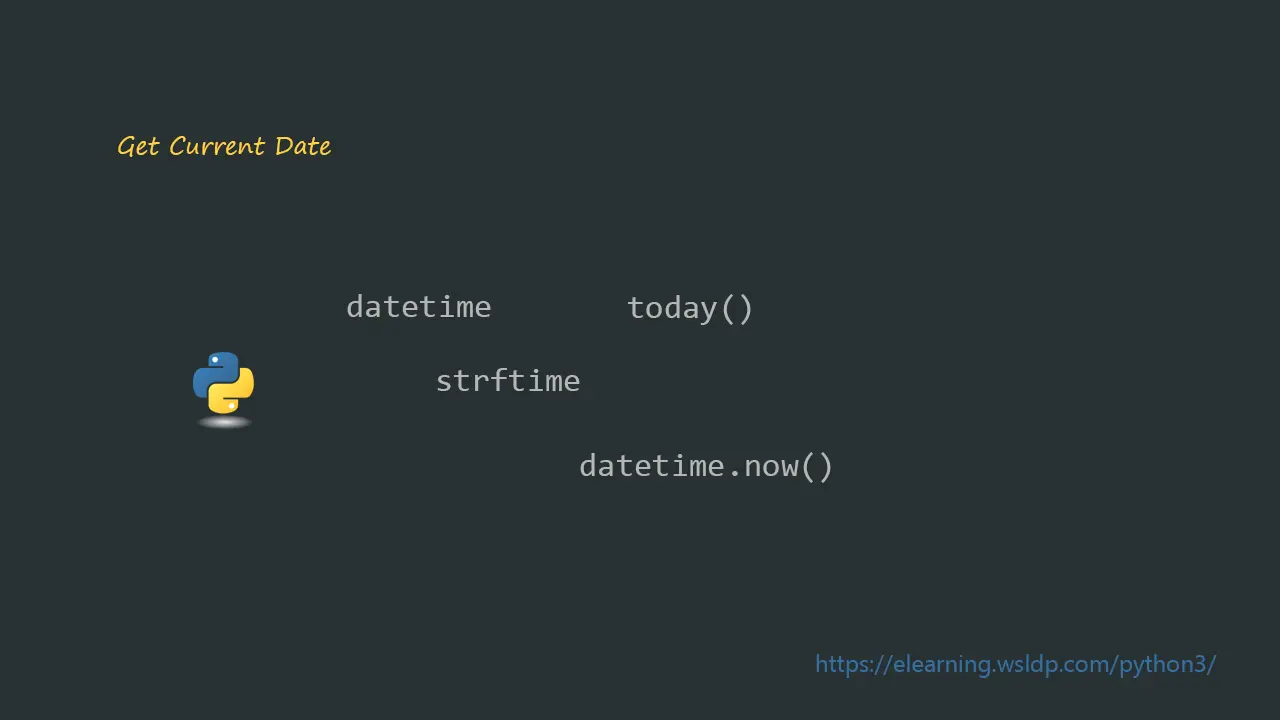
In This Python tutorial, you will learn how to get the current date in python 3. We will also learn how to get the current year, month, and day separately.
Get Current Date with Current Time in Python 3
To get the current date in python 3 we can use the datetime
module. First, we have to import the datetime
module in our python script.
#!/usr/bin/python3
import datetime
Then we can get the current date from the datetime
module using the date.today()
method.
#!/usr/bin/python3
import datetime
current_date = datetime.date.today()
print(current_date)
Above Python 3 script will output today’s date in year-month-date
format.
From the current date, we can also extract the current year, month, and day separately.
#!/usr/bin/python3
import datetime
current_date = datetime.date.today()
current_year = current_date.year # Extract current year only
current_month = current_date.month # Extract current month only
current_day = current_date.day # Extract current day only
Format Date with strftime function in Python 3
Python datetime module by default outputs the current date in year-month-date
format. But if we want we can use the strftime()
method to output the date in different formats.
Example 1
#!/usr/bin/python3
import datetime
current_date = datetime.date.today()
current_date = current_date.strftime("%d-%m-%Y")
print(current_date)
In the above example, we change the format of the current_date
to the date-month-year
format using the strftime()
function.
Example 2
#!/usr/bin/python3
import datetime
current_date = datetime.date.today()
current_date = current_date.strftime("%d-%B-%Y")
print(current_date)
In the above example, we display the Month as a full name(%B
) instead of the decimal number.
You can get the complete list of string formats used in the python strftime function from https://strftime.org/.
Get Current Date with Current Time
To get both the current date and time, we can use the datetime.datetime.now()
method.
#!/usr/bin/python
import datetime
date_time = datetime.datetime.now()
print(date_time.strftime("%Y-%b-%d %H:%M"))
As per the above example, first, we assigned today’s date and time to the date_time
variable. Then we displayed the date and time in year-month-date hour:minutes
using the strftime
function. The output will be something similar to the following.
2021-Aug-10 09:12
You can refer to the datetime documentation For more advanced python 3 datetime
functions.