How to Get Current Time in Python 3
We can get the current time in Python 3 using the time module of the Python’s standard library.
In the Python interpreter, first import the time module:
import time
Then run the following command which will print the current time 24-hour format:
time.strftime("%H:%M")
Print time in 12 Hour clock format:
time.strftime("%I:%M")
Get the current time with second:
time.strftime("%H:%M:%S")
The time module provides a way to retrieve the current date and time on a system. We can construct different time formats using string format. Following string format can be used to get current time along with the current date:
time.strftime("%Y-%b-%d %H:%M:%S")
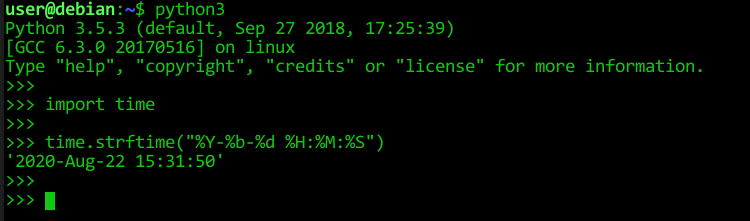
One can use the time module to get the current time in a Python script, as shown in the following Python 3 script:
import time
print(time.strftime("%H:%M"))
The output will be the current time in the 24 Hour Clock.
Using the string format, we can retrieve the current hour, minute, or even the current second separately.
Examples
Current Hour in as a zero-prefixed decimal number (e.g., 05):
print(time.strftime("%H"))
Get current Hour in as a decimal number (e.g., 5):
print(time.strftime("%-H"))
Current Hour in 12-hour clock with zero prefixed:
print(time.strftime("%I"))
Current Hour in 12-hour clock as a decimal:
print(time.strftime("%-I"))
Get the current minute:
print(time.strftime("%M"))
Second with zero prefixed:
print(time.strftime("%S"))
Current second as a decimal:
print(time.strftime("%-S"))