How to Get Current Year in Python 3
In this tutorial, we will learn how to get the current year in python 3 using the datetime module.
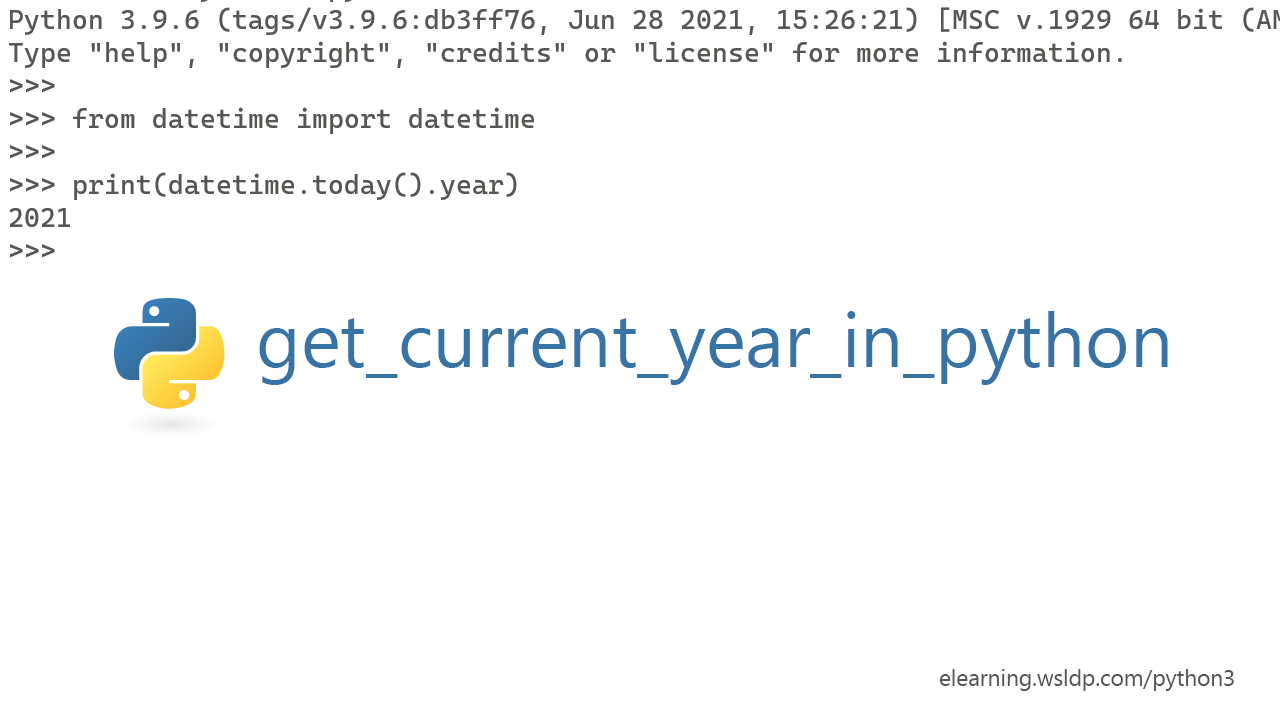
To get the current year, we need to import the datetime
submodule from the datetime
module.
from datetime import datetime
After importing the datetime
module, we can print the current year as follows:
from datetime import datetime
print(datetime.today().year)
If you want to get the current year in two-digit format, use the .strftime
method as follows:
print(datetime.today().strftime("%y"))
How it works…
In the earlier example, first, we imported the datetime
submodule, which is part of the datetime
module (both the main module and sub-module have the same name).
Python 3 datetime
submodule comes with a method called today()
, which holds information about the current time.
We want the current year, which we can get by appending .year
to the today()
method.
from datetime import datetime
print(datetime.today().year)
The same way we can get the current month, day, hour, minute, and second.
from datetime import datetime
current_year = datetime.today().year #Current Year
current_month = datetime.today().month #Current Month
current_day = datetime.today().day #Current Day
current_hour = datetime.today().hour #Current Hour
current_minute = datetime.today().minute #Current Minute
current_second = datetime.today().second #Current Second
In the above Python code, we stored the current year, month, and date in separate variables.