Learn How to Open Web Browser in Python Script
In this tutorial, we are going to learn how to open a web browser using python 3.
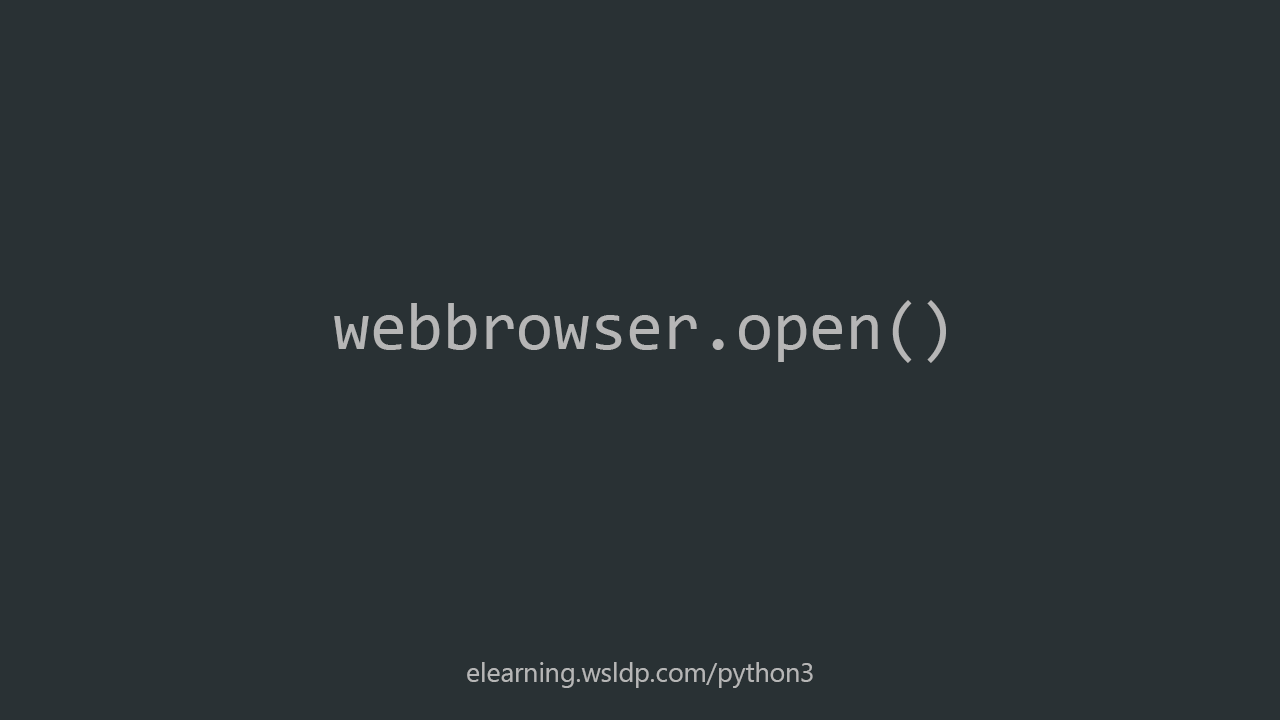
Python has a module called webbrowser
, which allows you to open the web browser from a python script by simply calling the open()
function of the module.
The webbrowser.open()
method will open your default web browser with a given URL.
Example
First, we need to import the python webbrowser
module using the import
statement.
import webbrowser
Then call the open()
function with the URL of the website as an argument.
import webbrowser
webbrowser.open("https://google.com")
Above Python 3 script will open the URL https://google.com
with the default web browser of the user’s computer.
Select the Web Browser
The webbrowser
module by default uses the system default web browser, but by combining the .open()
function with the .get()
function, we can open a different browser installed on the computer.
Example 1
import webbrowser
webbrowser.get("firefox").open("https://www.bing.com")
As per the above example, URL https://bing.com
will be open with Firefox web browser.
Example 2
import webbrowser
webbrowser.get("google-chrome").open("elearning.wsldp.com/python3/")
The above python script will open google chrome in Linux.
In Microsoft Windows, you will have to add the executable path of the web browser to the Windows PATH
variable, in order to get()
function to work.