Python Remove Element from List
In this tutorial, you will learn how to remove elements (items) from Python lists.
We are going to look at two built in list methods: .remove() and .pop().
Removing list elements by value with remove()
The remove()
function removes an element by its value. It takes the value of the item as its sole argument.
list.remove('value')
Let’s look at the code examples now:
languages = ['Java', 'PHP', 'C#', 'Python']
languages.remove('PHP')
print(languages)
In the preceding code example, the second element (PHP) is removed from the list by the remove()
function.
If there are multiple occurrences of a given value, the remove()
method removes the first occurrence of that value from the list. For example, look at the following Python code:
languages = ['Java', 'PHP', 'C#', 'Python', 'Java']
languages.remove('Java')
print(languages)
In the preceding example, the list has two occurrences of “Java”, but only the first from the left is removed by the languages.remove('Java')
.
If the value is not in the list, Python will raise an error:
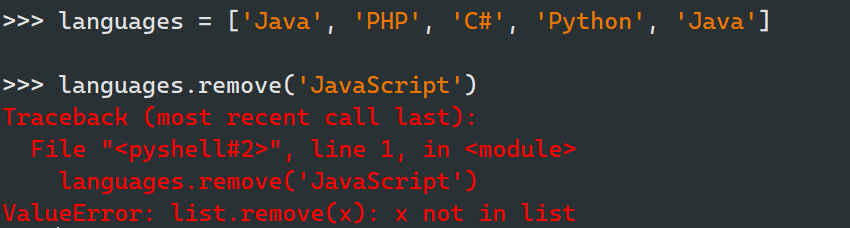
To avoid this error, you can run the remove()
function inside a try block, as shown in the following example:
languages = ['Java', 'PHP', 'C#', 'Python', 'Java']
try:
languages.remove('JavaScript')
except:
print("The item was not found in the list.")
else:
print("The item was removed.")
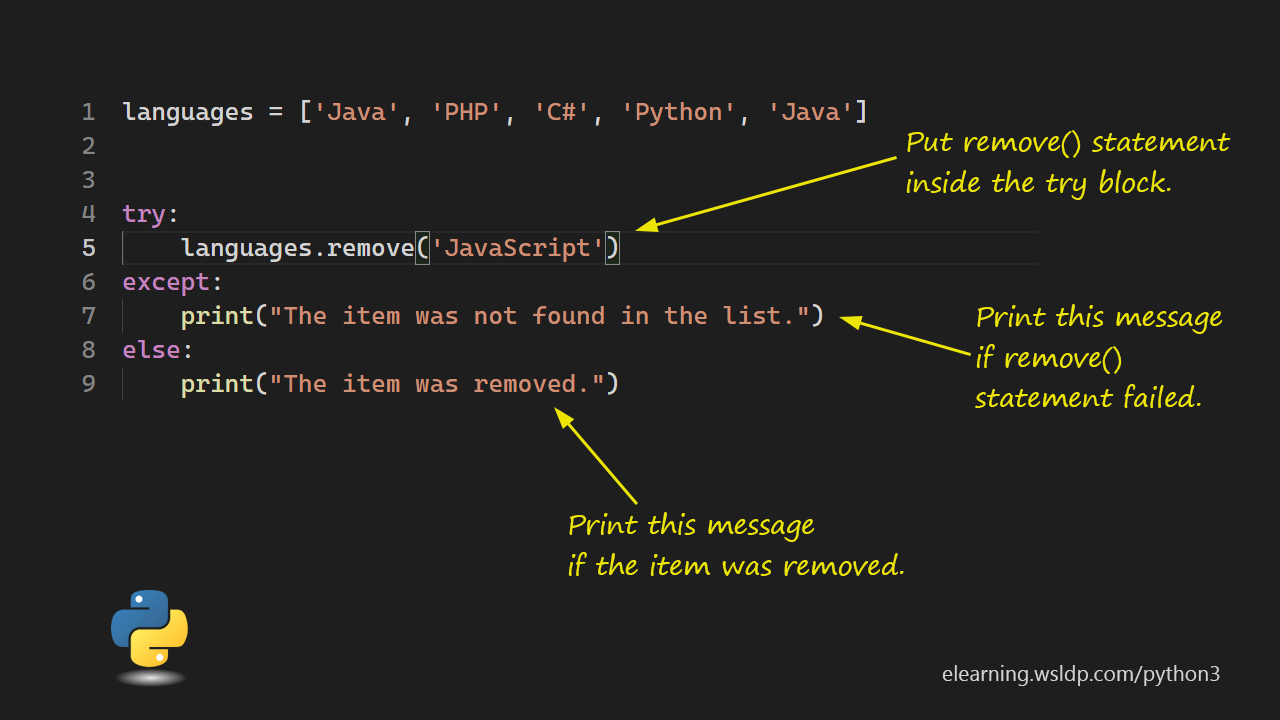
Removing list elements by index with pop()
It’s also possible to remove elements by index position rather than by value, using the pop()
method.
The pop()
function removes an element by its index position. If you run the pop()
method without specifying an index value, the last item in the list is removed and returned.
For example, consider the following example:
languages = ['Java', 'PHP', 'C#', 'Python']
languages.pop(0)
print(languages)
In the preceding example, the pop()
function in Python removed the 0 index position, which is the first item in the list.
Following python script invokes pop without providing an index value. Therefore, the last element in the list is removed and returned.
languages = ['Java', 'PHP', 'C#', 'Python']
languages.pop()
print(languages)
Note that the pop()
function removes and returns the element. What that means is that the item removed by pop()
can be assigned to a variable, as shown in the following code example:
languages = ['Java', 'PHP', 'C#', 'Python']
removed = languages.pop(0)
print(languages)
print(removed)
If the list is empty or the index position does not exist, the pop function in Python raises an error. Again to avoid this situation, run the pop()
function inside a try block.