How to Remove First Element from List in Python 3
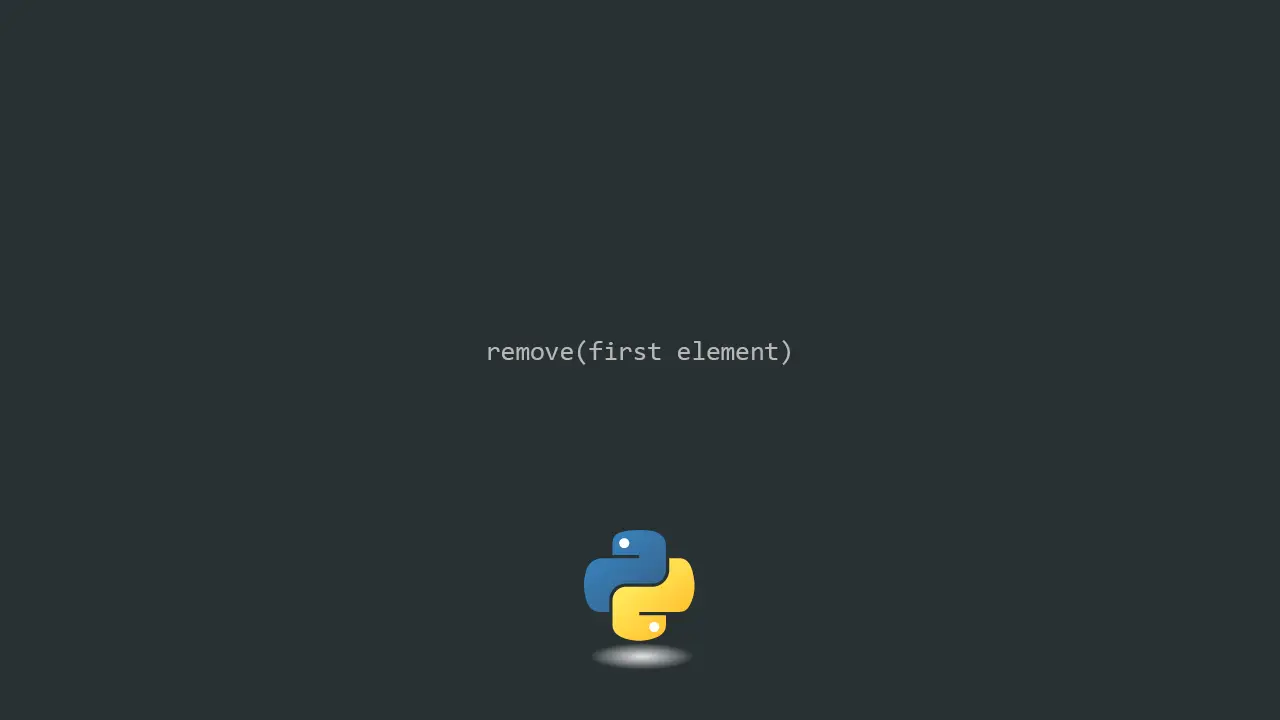
In Python 3, to remove the first element from a list, we can use either the pop()
function or del
statement. Both methods remove elements from lists by index position.
The index of the first element is always 0
Here is an example where we use the pop function to remove the first element from a list:
numbers = [1, 2, 3, 4, 5]
numbers.pop(0)
If you want, you can remove the first item and store it in a variable:
numbers = [1, 2, 3, 4, 5]
removed = numbers.pop(0)
print(removed)
print(numbers)
If you don’t specify an index value in the pop()
function, it removes the last element from the list:
numbers = [1, 2, 3, 4, 5]
removed = numbers.pop()
print(removed)
print(numbers)
The del
statement is another method of deleting list items in Python 3. Just like the pop
function, the del
method also deletes list items by index position.
The following code shows how the del
method works to remove the first element from a list:
numbers = [1, 2, 3, 4, 5]
del numbers[0]
The del
method does not return the value, unlike the pop()
function.