Python Remove Last Element From List
To remove the last element of a Python list, use the pop()
function. For example, consider the following example:
car_list = ['Ferrari', 'Porsche', 'Bugatti', 'Lamborghini']
car_list.pop()
print(car_list)
In the example, the pop()
method will remove the last element (Lamborghini) from the car_list
.
The pop method removes and returns the last element from the list. This means items removed by pop can be assigned to a variable if you want.
For example, the following example pops the last element from the car_list
list and then assign the value of that element in a new variable called removed
:
car_list = ['Ferrari', 'Porsche', 'Bugatti', 'Lamborghini']
removed = car_list.pop()
print(car_list)
print(removed)
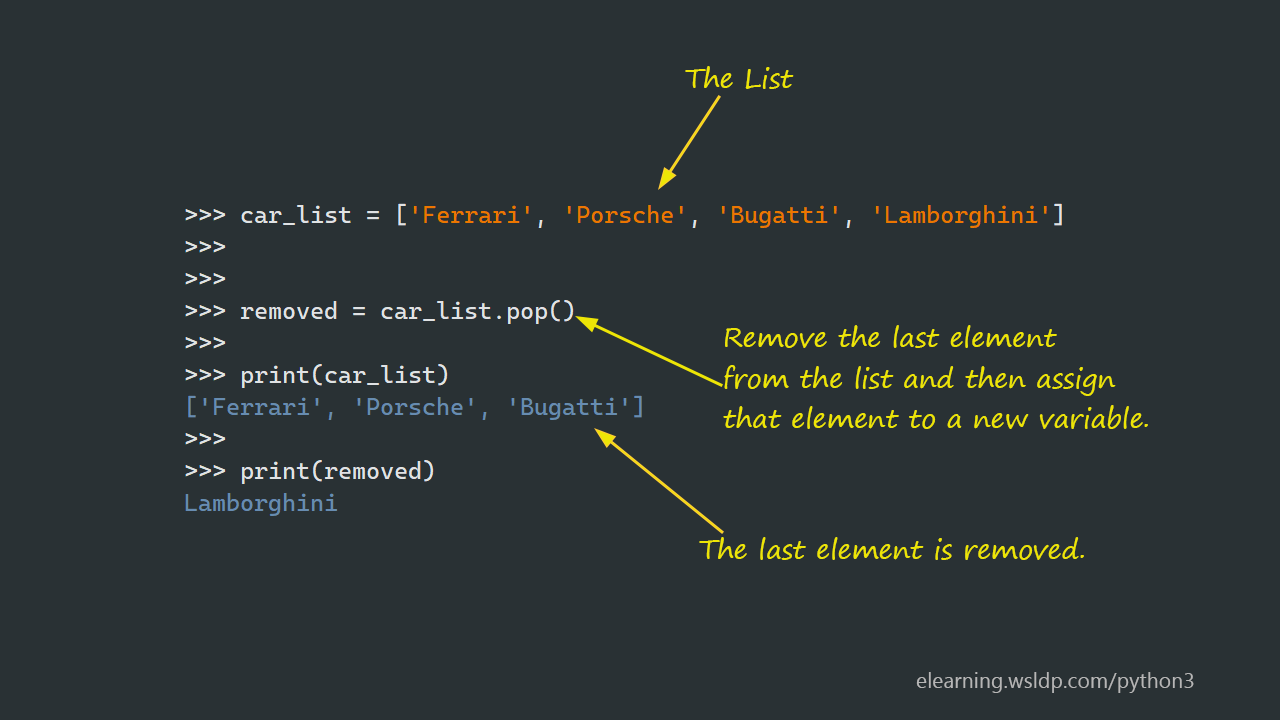
The pop()
function in Python deletes items from a list by index. If an index is not provided with the pop()
function, the last element in the list is removed.
If you specify an index position, the item in that position is removed and returned.
The following code snippet demonstrates the use of Python pop()
with an argument:
car_list = ['Ferrari', 'Porsche', 'Bugatti', 'Lamborghini']
car_list.pop(0)
print(car_list)
The above example deletes the first item from the car_list list. Zero(0) corresponds to the first item in the list.