How to Reverse a List in Python Programming
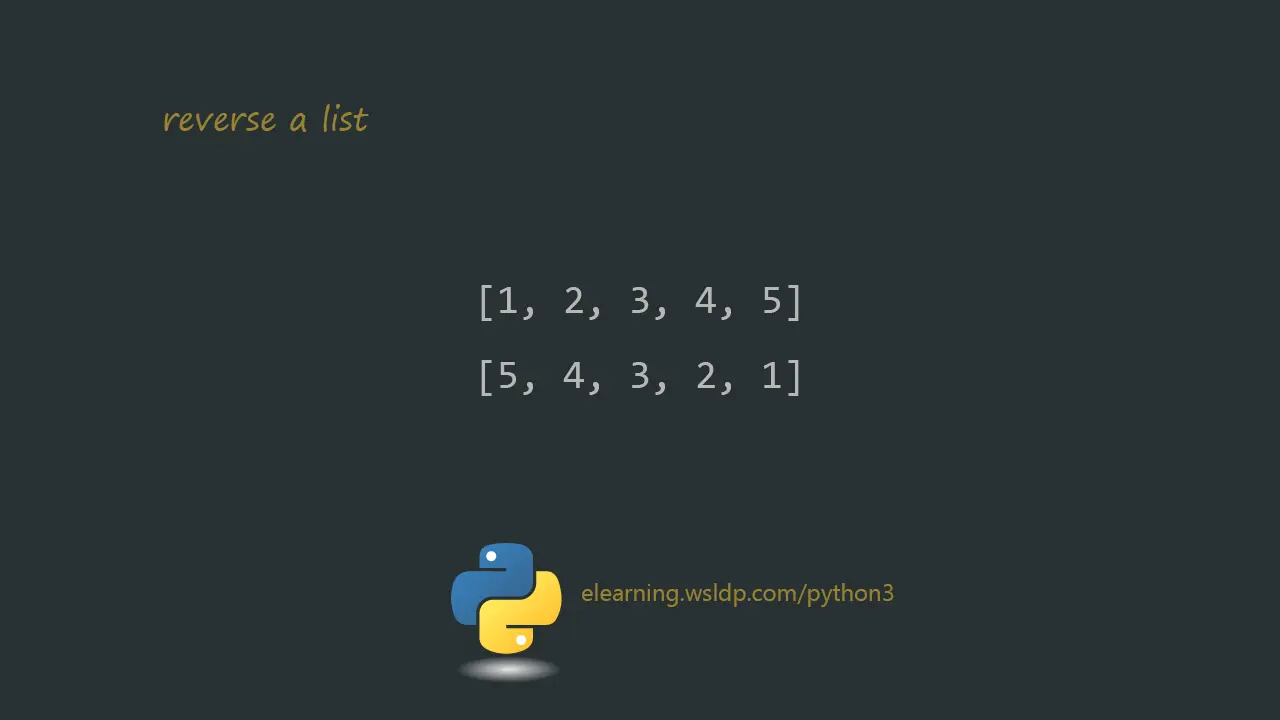
Python 3 comes with a built-in list method called reverse()
that reverses an existing list.
num = [1, 2, 3, 4, 5]
print(num)
num.reverse()
print(num)
If we run the preceding code, we get the following output:
[1, 2, 3, 4, 5]
[5, 4, 3, 2, 1]
Reverse a list without changing the original list
The reverse method alters the existing list. If you want to reverse a list without changing the original list, you will want to use the square bracket notation (slicing).
The following code example shows how to reverse a list in Python using square bracket notation:
num = [1, 2, 3, 4, 5]
print(num[::-1])
In the previous example, we print the list in reverse order without changing the original list.
Here is another example without using the reverse function:
num = [1, 2, 3, 4, 5]
num_reverse = num[::-1]
print(num)
print(num_reverse)
In this example, we reverse the num list using the square bracket notation. We also store the reverse list in a new variable called num_reverse
.
The following code shows how to use for loop to iterate through a reverse list in Python:
num = [1, 2, 3, 4, 5]
for i in num[::-1]:
print(i)
The syntax of the Python square bracket notation (slicing) is list(start, stop, step_count)
.
When reversing a list, we omit the start and stop index to get all list items. Then we put -1
in the third field to reverse the list.