Python List Insert – Add Element to List at Index
Previously, we learned the append and extend methods to insert items to list in Python.
But both those methods add items to the end of an existing list and cannot be used to insert items at a specific index position.
When you want to insert an item at a specific index position, you’ll want to use the insert()
method.
The general syntax of insert()
is as follows:
list.insert(index, element)
The insert
method takes an index value as its first argument and an element as its second argument.
The following Python code shows how it works:
countries = ['Brazil', 'Colombia', 'Denmark','Fiji']
countries.insert(0, 'Argentina')
In this example, we inserted a new item to the beginning of the list (at index 0) called countries (Python uses zero-based indexing).
We can use any index position, following is another example:
countries = ['Brazil', 'Colombia', 'Denmark','Fiji']
countries.insert(0, 'Argentina')
print(countries)
countries.insert(4, 'Ecuador')
print(countries)
In the preceding example, we inserted Ecuador into the Python list at the fourth index position.
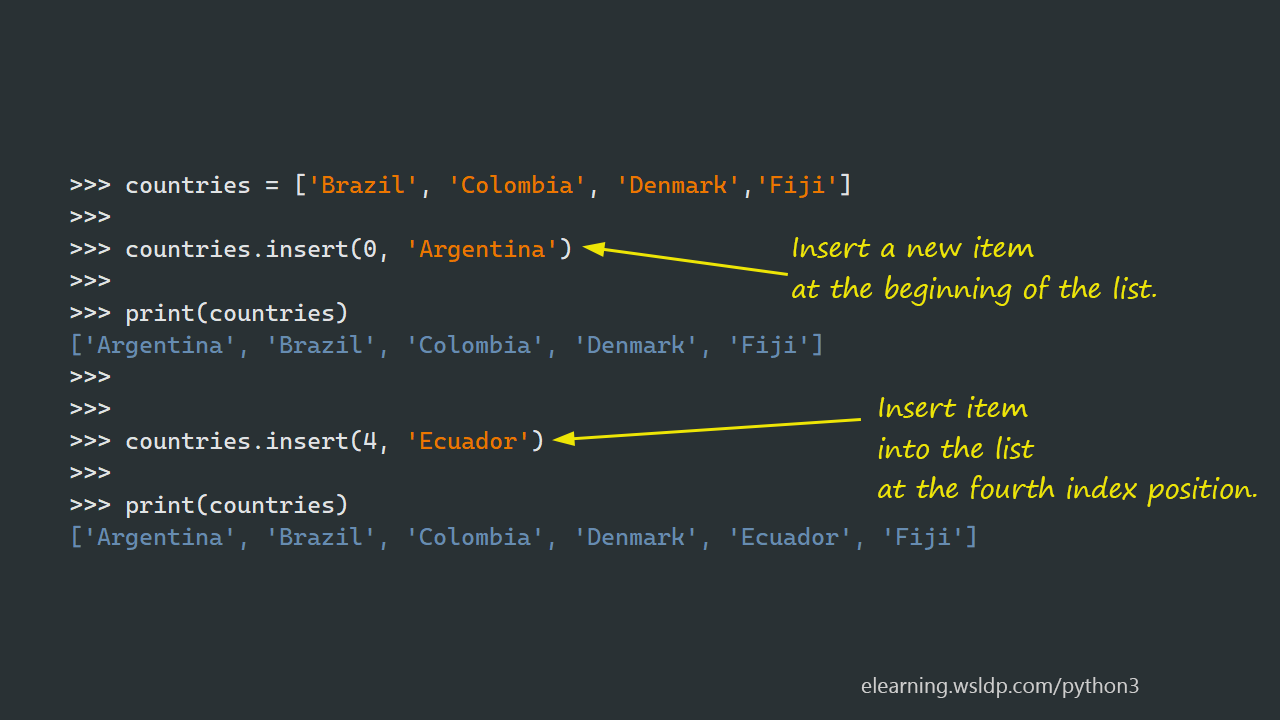